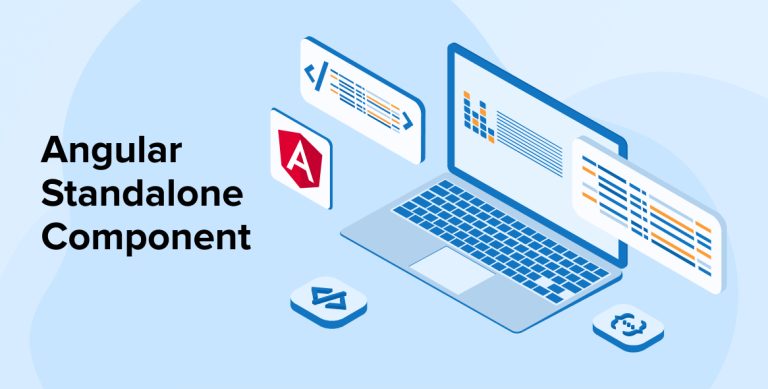
Angular is a powerful framework that breaks the conventional boundaries to enhance the software development process. Every new version release of Angular offers some major advancements that developers along with the whole industry eagerly anticipate.
Key features from the latest releases include server-side rendering, improved reactivity, enhanced tooling, and standalone APIs. Leading Angular development companies are well aware of how to leverage these features for maximum benefit.
This blog focuses on Angular standalone components and discusses how they can be transformative for your project. Let us understand the concept in detail along with its use cases.
1. What is a Standalone Component?
A type of component in Angular that operates independently of any Angular module. Before Angular 14 was released, developers had to declare components and pass them inside the module’s declaration array. Otherwise, Angular would throw an error and cease to compile.
But with the release of Angular 14, it has become possible to create standalone components that aren’t part of any module. Now, Angular also allows you to create standalone pipes and directives.
Further Reading on:
Angular Pipes
Directives in Angular
2. How to Declare a Standalone Component?
You need to take some preparatory steps. Refer to the example given below to understand how you can declare a standalone component that doesn’t have to be dependent on any module of your Angular application.
import { CommonModule } from '@angular/common'; import { Component, Input } from '@angular/core'; @Component({ selector: 'demo-image-viewer', standalone: true, imports: [CommonModule], template: `<div class="col-md-4"> @for(imgUrl of images; track imgUrl){ <img [src]="imgUrl" /> } </div>`, styles: ``, }) export class DemoImageViewerComponent { @Input() images = []; } |
3. How to Create an Angular Standalone Component?
It is very easy to create a standalone component, directive, or pipe. All you have to do is mark it with:
standalone: true |
In Angular 14, there isn’t any CLI command available that enables you to create a standalone component. So, in there, you have to add the flag yourself. However, all the versions from Angular 15 have CLI commands that help you generate a standalone component.
ng g component --standalone |
In the component’s decorator, add the standalone flag. But do not declare the Angular classes with this flag in your NgModules’ declarations array. Because doing so would result in an error in the Angular compiler.
Use the code example below to understand how you can add a standalone flag in your components, directives, or pipes.
import { Component } from '@angular/core'; import { DemoImageViewerComponent } from '../image-viewer/image-viewer.component'; import { CommonModule } from '@angular/common'; @Component({ selector: 'demo-photo-gallery', standalone: true, imports: [CommonModule, DemoImageViewerComponent], template: `<div class="photo-gallery mt-5"> <div class="row"> <demo-image-viewer [images]="userProfile.images"></demo-image-viewer> </div> </div>`, styles: ``, }) export class DemoPhotoGalleryComponent { userProfile: any = {}; } |
You can see that the above code declares an import array in the component decorator. Now, import all the dependencies you need in the imports array to make your standalone component work. Any other standalone components, directives, and pipes you want to use should also be imported here.
It is true that not every standalone component, directive, and pipe you use will bear a standalone flag. They might have been declared in the module file instead. In such an instance, just import those modules into your standalone component and use the required components, directives, and pipes through that module.
import { Component } from '@angular/core'; import { DemoImageViewerComponent } from '../image-viewer/image-viewer.component'; import { CommonModule } from '@angular/common'; import { MatButtonModule } from '@angular/material/button'; @Component({ selector: 'demo-photo-gallery', standalone: true, imports: [CommonModule, DemoImageViewerComponent, MatButtonModule], template: `<div class="photo-gallery mt-5"> <div class="row"> <demo-image-viewer [images]="userProfile.images"></demo-image-viewer> </div> <button mat-button (click)="submit()">Submit</button> <button mat-fab (click)="cancel()">cancel</button> </div>`, styles: ``, }) export class DemoPhotoGalleryComponent { userProfile: any = {}; submit() { // logic to submit user profile details } cancel() { // Navigate back to last page } } |
No matter if those modules are internal or from third-party libraries, after importing them, you can use their components. An example of the same is given in the code above.
4. How to Utilize a Standalone Component
By now, you have learned what is a standalone component and how to create them. Now, it’s time you learn how to use them. Well, there are certain use cases as follows:
4.1 In Another Standalone Component
When you need to use your standalone component in another one, just pass it to another standalone component’s imports property. The code given below shows exactly how you should do it.
// my-standalone.component.ts import { Component, OnInit } from "@angular/core"; Import { CommonModule } from ‘@angular/common’; Import { MyHomeComponent } from ‘../my-home/my-home.component’; @Component({ Selector: ‘app-my-standalone’, Standalone: true, Imports: [CommonModule], templateUrl: ‘./my-standalone.component.html’, styleUrls: [‘./my-standalone.component.css’] }) Export class MyStandaloneComponent implements OnInit { constructor() { } ngOnInit(): void { } }’ |
Now you can use another standalone component on the standaloneComponent as below:
// my-standalone.component.html <h3> Welcome to My Standalone Component </h3> <app-my-home> </app-my-home> |
4.2 Inside a Module
First, create a new module-based project and add your standalone component to it. The source code will look like this:
//tatva-footer.component.ts import { Component } from '@angular/core'; @Component({ selector: 'tatva-footer', standalone: true, imports: [], template: `<p class="copyright">V 1.1.0 | ©{{ currentYear }} Demo Tatvasoft. All Rights Reserved.</p>`, styleUrl: './tatva-footer.component.scss', }) export class TatvaFooterComponent { currentYear: number; constructor() { this.currentYear = new Date().getFullYear(); } } |
What if we want to see the standalone component; the tatva footer in our AppComponent?
Being a part of the AppModule, the AppComponent isn’t a standalone component. Just import the TatvaFooterComponent to the imports metadata array to use it in the AppComponent.
//app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { AppComponent } from './app.component'; import { TatvaFooterComponent } from './tatva-footer.component'; @NgModule({ imports: [BrowserModule, FormsModule, TatvaFooterComponent ], declarations: [AppComponent], bootstrap: [AppComponent], }) export class AppModule {} |
Next, you have to render the TatvaFooterComponent after opening the app.component.ts.
// app.component.ts import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <h1>Hello from {{ name }}!</h1> <tatva-footer></tatva-footer> `, }) export class AppComponent { name = 'App Component'; } |
With the execution as mentioned above, you can start using your standalone component in a module.
4.3 Using Standalone Components Combined with NgModules
NgModules are used excessively for various purposes, like managing lazy loading and code splitting. For that, you can use the Angular standalone component in a NgModule-based app.
Add the Angular module consisting of a standalone component to the NgModule decorator. Register all the standalone components in the imports array and declare regular components in the declaration array. This is required when you have to utilize a standalone component hailing from the component declared in a NgModule.
@NgModule({ declarations: [TatvaFooterComponent ], exports: [TatvaFooterComponent ], imports: [TatvaFooterComponent ], <-- standalone component }) export class SharedModule {} |
5. Lazy Loading with Standalone Components
Lazy Loading becomes very easy with the use of standalone components. Initially, developers were using modules for lazy loading. And they had to create a separate module for every set of directives, pipes, or components that required lazy loading.
The entire process was overwhelming and needed a lot of boilerplate. Developers also had to configure the modules in the router and manually import all the dependencies. Below is an example of how the routing configuration of a module with lazy loading looks:
const routes: Routes = [ { path: "one", loadChildren: () => import("./module-lazy/modulelazy.module"). then((m) => m.ModuleLazyModule), }, ]; |
The routing configuration is not responsible for the overhead in the lazy loading of modules. It is borne out of the need to create a module and define all of its dependencies. So, your wanting to cover some specific features to make them reusable is not the reason. However the very process of lazy loading the content leads to overhead.
However, things are completely different when you use standalone components:
export const ROUTES: Route[] = [ { path: "user-profile", loadComponent: () => import("./user-profile") .then((m) => m.UserProfileComponent), }, ]; |
In this new lazy loading process, just pick the loadComponent option and point to the route’s high-level container. And your work is done here. This process doesn’t demand you to create a separate module for every screen that you lazy load. It also doesn’t ask you to import the necessary dependencies those screens need.
Lazy loading is no longer an overwhelming process if you use standalone components. It becomes easy.
6. How to Bootstrap Angular Standalone Components?
Your code will look like the snippet given below when, instead of using NgModules, you bootstrap the app with Standalone Component APIs.
import { bootstrapApplication } from "@angular/platform-browser"; bootstrapApplication(MyStandaloneComponent); |
The standalone component that you want to turn into the app’s root is passed through the bootstrapApplication API. It also helps you import any dependency you need, including forms and routers. In short, app bootstrapping becomes easy with standalone components.
7. Benefits of Standalone Components
Developers prefer to use standalone components to enjoy a multitude of benefits, including:
7.1 Reusability
With standalone components, you can encapsulate every app functionality into a separate self-contained unit. Such units are easy to reuse in various projects. This helps save time and reduce code duplication.
7.2 Portability
Standalone components are highly portable since they are independent of any app or module. Hence, developers don’t face any difficulties in sharing and distributing them. Moreover, these standalone components are packaged and distributed as libraries through NPM and other package managers. This allows developers to add specific functionalities to their applications.
7.3 Simplified Maintenance
When you turn an app functionality into a standalone unit, the maintenance of its codebase won’t be complex anymore. It’s easy to make changes or update a standalone component. Because it is independent, you only have to implement the same changes once. And when it is used with the app, the changes will reflect across the entire application. This helps simplify the maintenance of the application and its codebase.
7.4 Improved Collaboration
Standalone components promote the use of a standardized method to create and share reusable UI elements. Because of that, developers have to collaborate to create a library of shared components that can be helpful in various projects. Such collaboration also helps improve efficiency and consistency.
7.5 Enhanced Testing
Testing the self-contained units individually is more efficient. You can quickly identify the bugs and fix issues in the code, resulting in increased reliability of standalone components.
7.6 Modularity
Standalone components encourage the modular approach to app development. Here, when you break down a large app into small manageable pieces, you not only reduce its complexities but also make it easily maintainable.
8. Conclusion
Standalone components are basically small and independent modules of your application. That is why you can easily reuse it across different projects or in the same application as well.
There are many more benefits of using such components. Developers can effectively leverage them to increase the scalability and efficiency of their Angular applications. Moreover, using them helps reduce the load times and enhance runtime performance. As a result, you get to build a responsive application.
Moreover, there is no hassle in updating or modifying the code of standalone components. Hence, the maintainability of your codebase and application won’t be an issue anymore. In short, you can say that the use of standalone components helps streamline the entire app development process, which eventually leads to the success of your project.
FAQs
What are Angular standalone components?
The type of components that aren’t required to be declared in any module of an Angular application and function independently are called Angular standalone components. They are either used directly from the template of another component or are imported to any NgModule.
What is lazy loading in Angular?
When you allow dynamic loading of specific modules of your Angular application, it is called lazy loading. These modules are only loaded when users navigate a specific route associated with them. Lazy loading is an effective technique for reducing the initial loading time.
Comments
Leave a message...