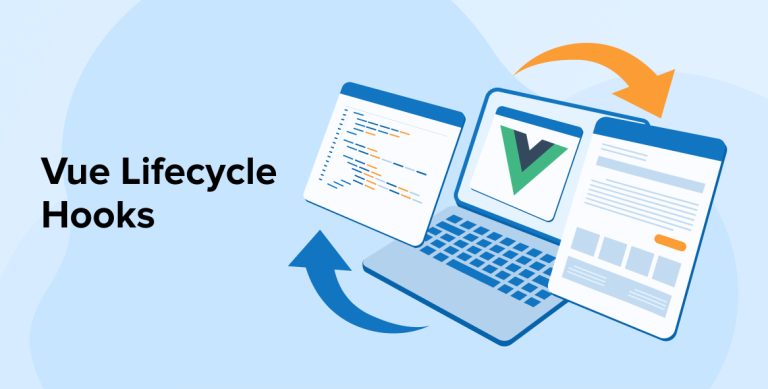
When it comes to front-end development, Vue is a popular choice. It has strong community support that actively contributes to the advancement of the framework over time. This is why successive versions of Vue become increasingly efficient and equipped with powerful features. As a result, a top VueJS development company can easily deliver responsive and dynamic web applications. In this article, we will discuss one of the most robust features of Vue called lifecycle hooks. They are used to perform specific actions at different stages of a component’s lifecycle.
Here, we will explore what lifecycle hooks are, their types, and how they are used in real-world scenarios.
1. What are Vue Lifecycle Hooks?
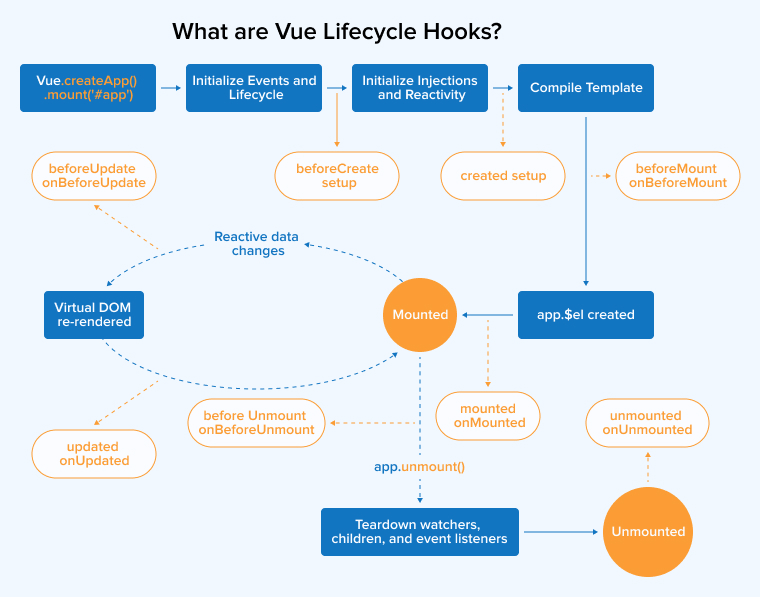
Lifecycle hooks are special methods involved automatically by Vue at specific stages of a component’s lifecycle, from its creation to deletion. You can use them to run custom logic at different stages of a Vue instance’s lifecycle.
Although their behavior is similar to events, lifecycle hooks in Vue are specifically bound to the components rather than user interactions. If you want to gain insight into a component’s lifecycle stages such as when they are created, updated, or deleted, then Vue’s lifecycle hooks are the ideal choice.
There are lifecycle hooks for every stage of a component’s lifecycle, and we will discuss them in detail, along with suitable examples.
2. Understanding Creation Hooks
The first Vue hook that can be executed in your component is the creation hook. They are run before a component is added to the DOM. It allows you to perform specific actions or configure settings during server-side or client-side rendering.
Creation hooks are the only Vue lifecycle hooks that can be executed during server-side rendering.
2.1 beforeCreate Hook
The beforeCreate hook is invoked before a Vue instance or component is initialized. At this point, events, computed properties, data, and other stages have not yet been set. The beforeCreate hook offers an early entry point to perform setups or specific actions before initialization.
Let’s take a look at an example to understand how it works:
<script> export default { beforeCreate() { console.log('Before Create Lifecycle Hook: Instance is being created') }, } </script> |
The code above calls the beforeCreate lifecycle hook before fully initializing the Vue instance. This enables the developers to log information or take specific actions before setting up options for components, events, or data observations. In this example, the Vue hook logs a message in the console, indicating that the instance creation process has begun.
2.2 created Hook
The created lifecycle hook runs after the initialization of the Vue instance but before mounting or rendering the templates and Virtual DOM. At this stage of the Vue component’s lifecycle, the component instance has processed all state-related options.
This allows you to access active events, computed properties, and reactive data. Additionally, you can perform further setups. Below is a working example of the created hook:
<template> <div> <p>Weather Dashboard for {{ cityName }}</p> <p v-if="isWeatherDataLoading">Loading data for Weather Dashboard</p> <div v-else> <strong>Place: {{ weatherDataForCity.place || 'City Not Fetched'}}</strong> <br /> <strong>Temp: {{ weatherDataForCity.temp || 'Temp. Not Fetched' }}</strong> </div> </div> </template> <script> export default { name: 'LifeCycleComponent', data() { return { cityName: '', isWeatherDataLoading: false, weatherDataForCity: { place: 'Bodakdev', temp: 18 } } }, computed: { cityComputed() { return this.cityName; } }, beforeCreate() { console.log('Before Create Lifecycle Hook: Instance is being created'); }, created() { console.log('Created Lifecycle Hook: City is reactive and it will update'); this.cityName = 'Ahmedabad'; } } </script> |
Here, we have a LifeCycleComponent with a weather dashboard for a specific city. It displays the weather details and loading messages using conditional rendering based on the isWeatherDataLoading flag. After creating the component instance, we use the created lifecycle hook to initialize the cityName property to “Ahmedabad”. This will ensure the data is reactive and ready for rendering once the template is processed.
3. Understanding Mounting Hooks
These are the most widely used lifecycle hooks. With Mounting hooks, you can access the component both before and after its first rendering to modify the DOM. However, they can’t be executed during server-side rendering.
3.1 beforeMount Hook
The beforeMount hook is called right before the initial rendering or mounting of the component to the DOM. It can also be invoked after compiling the render functions or templates. It is useless to access the DOM element through the beforeMount hook, as they are not accessible until a component is added to the DOM. So, you can use this lifecycle hook to perform actions before updating the DOM.
<template> <div> <p>Weather Dashboard for {{ cityName }}</p> <p v-if="isWeatherDataLoading">Loading data for Weather Dashboard</p> <div v-else> <strong>Place: {{ weatherDataForCity.place || 'City Not Fetched' }}</strong> <br /> <strong>Temp: {{ weatherDataForCity.temp || 'Temp. Not Fetched' }}</strong> </div> </div> </template> <script> export default { name: 'LifeCycleComponent', data() { return { cityName: '', isWeatherDataLoading: false, weatherDataForCity: { place: 'Bodakdev', temp: 18 } } }, computed: { cityComputed() { return this.cityName; } }, beforeCreate() { console.log('Before Create Lifecycle Hook: Instance is being created'); }, created() { console.log('Created Lifecycle Hook: City is reactive and it will update'); this.cityName = 'Ahmedabad'; }, beforeMount() { console.log('Before Mount Lifecycle Hook: Component is about to be mounted'); } } </script> |
The LifeCycleComponent in Vue is responsible for rendering a weather dashboard that either displays a loading message or shows weather details for a specific city, based on the isWeatherDataLoading flag. A message is logged using this Vue lifecycle hook before adding the component to the DOM to confirm that the template is well-compiled and ready to be mounted.
3.2 Mounted Hook
Once the component is rendered and added to the DOM, you can use the mounted hook, which is called after the component is mounted, to perform actions like setting up event listeners or fetching external data. This is the first point in a Vue instance’s lifecycle where you can interact with the DOM elements. By using the mounted hook, you can access the rendered DOM, templates, and reactive components.
<template> <div> <p>Weather Dashboard for {{ cityName }}</p> <p v-if="isWeatherDataLoading">Loading data for Weather Dashboard</p> <div v-else> <strong>Place: {{ weatherDataForCity.place || 'City Not Fetched' }}</strong> <br /> <strong>Temp: {{ weatherDataForCity.temp || 'Temp. Not Fetched' }}</strong> </div> </div> </template> <script> export default { name: 'LifeCycleComponent', data() { return { cityName: '', isWeatherDataLoading: false, weatherDataForCity: { place: 'Bodakdev', temp: 18 } } }, computed: { cityComputed() { return this.cityName; } }, beforeCreate() { console.log('Before Create Lifecycle Hook: Instance is being created'); }, created() { console.log('Created Lifecycle Hook: City is reactive and it will update'); this.cityName = 'Ahmedabad'; }, beforeMount() { console.log('Before Mount Lifecycle Hook: Component is about to be mounted'); }, mounted() { console.log('Mounted Lifecycle Hook: Component has been mounted'); this.isWeatherDataLoading = true; this.weatherDataForCity = { place: 'Maninagar', temp: 16 }; this.isWeatherDataLoading = false; } } </script> |
We are developing a LifeCycleComponent that displays a weather dashboard for a specified city, showing either the weather details or a loading message. Once the component is mounted to the DOM, we will use the mounted lifecycle hook to log a message.
Toggling the isWeatherDataLoading flag in this hook allows the Vue component to simulate a data-loading process. This enables the component to update the weather data and display weather details for the new location, in this case, Maninagar. Such dynamic data reactivity is possible after the component is mounted to the DOM.
4. Understanding Update Hooks
Data changes are obvious when you have full-functioning software in a production environment. The update stage in a component’s lifecycle addresses data modifications. Let’s take a look at the Vue lifecycle hooks used in this stage.
4.1 beforeUpdate Hook
Whenever a component’s data changes, the beforeUpdate hook is called before updating the DOM. A component needs to be re-rendered and patched when data changes occur. But before the DOM is updated, developers have the opportunity to work with existing DOM to perform actions such as removing event listeners using this hook.
Vue calls this hook right before the DOM tree is updated. The beforeUpdate hook allows you to access the DOM state. While running this hook, it is possible to implement modifications to the component state.
<template> <div> <h1>Runs Scored</h1> <p id="score">Current Score: {{ runs }}</p> <button @click="singleRunTaken">Single</button> </div> </template> <script> export default { name: 'ScoreComponent', data() { return { runs: 0, }; }, methods: { singleRunTaken() { this.runs++; }, }, beforeUpdate() { console.log('Before Update Lifecycle Hook: Score is about to update.'); console.log('Score value before DOM update:', document.getElementById('score').innerText); }, }; </script> |
In the above example, we have a game with its score tracked and displayed using a Vue component named ScoreComponent. The users can increase the game score by simply clicking a button, which updates the value, represented as runs.
Every time a change is detected in the reactive runs property, the beforeUpdate lifecycle hook is called. This hook logs a message indicating that the DOM is about to be updated. From this example, we can see how to use the beforeUpdate hook to observe and debug changes in the DOM state before re-rendering occurs.
4.2 updated Hook
The updated hook is called once the component’s template has been re-rendered and updated. It is important to note that this hook will not be invoked when the component is rendered for the first time, but only during subsequent re-renderings.
The Vue components need re-rendering when data changes occur. So, after updating the DOM, the updated lifecycle hook helps you take the necessary actions. But do not update the DOM inside the updated hook because it requires further renderings.
<template> <div> <h1>Runs Scored</h1> <p id="score">Current Score: {{ runs }}</p> <button @click="singleRunTaken">Single</button> </div> </template> <script> export default { name: 'ScoreComponent', data() { return { runs: 0, }; }, methods: { singleRunTaken() { this.runs++; }, }, beforeUpdate() { console.log('Before Update Lifecycle Hook: Score is about to update.'); console.log('Score value before DOM update:', document.getElementById('score').innerText); }, updated() { console.log('Updated Lifecycle Hook: Score has been updated'); console.log('New Score:', document.getElementById('score').innerText); }, }; </script> |
We have a ScoreComponent in our Vue app that updates and displays the score in real time during the game. Users can increase the score by clicking a button. After updating the reactive runs property and re-rendering the DOM, the updated lifecycle hook is called.
It logs the message to display the updated score. Here, the hook reads the DOM to log the new score value. In this way, the updated Vue lifecycle hook verifies or responds to changes in the DOM.
5. Destruction Hooks
These hooks are used at the last stage of the Vue instance’s lifecycle. They are triggered to perform specific actions when the component is destroyed or about to be.
5.1 beforeDestroy Hook
The beforeDestroy hook runs before your component is destroyed – you can use this hook to perform cleanup tasks or manage resources, such as removing event listeners, timers, subscriptions, or canceling network requests. The Vue instance is still fully functional at this stage. This hook is called to carry out the pre-destruction cleanup.
ScoreComponent.vue
<template> <div> <h1>Runs Scored</h1> <p id="score">Current Score: {{ runs }}</p> <button @click="singleRunTaken">Single</button> </div> </template> <script> export default { name: 'ScoreComponent', data() { return { runs: 0, }; }, methods: { singleRunTaken() { this.runs++; }, }, beforeDestroy() { console.log('Before Destroy Lifecycle Hook'); console.log('Final chance to access the DOM'); }, }; </script> |
In our App.vue component, we have a button that toggles the visibility of the ScoreComponent, which updates and displays the scored runs. In instances such as when the user clicks on the “Hide Score Component” button, then it triggers the beforeDestroy lifecycle hook before the component is removed from the DOM.
The log message from the beforeDestroy hook indicates that the component is about to be destroyed, indicating that the user has a last chance to access the DOM for necessary cleanups. This hook manages tasks such as logging information or releasing resources before the component is unmounted from the DOM.
App.vue
<template> <div id="app"> <button @click="show = !show">Show/Hide Score Component</button> <div v-if="show"> <ScoreComponent /> </div> </div> </template> <script> import ScoreComponent from './ScoreComponent.vue'; export default { components: { ScoreComponent }, data() { return { show: true }; }, }; </script> |
After hiding the component from the App.vue page, you can still see the logs from the beforeDestroy hook.
5.2 Destroyed Hook
As the last hook of the Vue instance’s lifecycle, the destroyed hook is called after a component is destroyed. Since the Vue component doesn’t exist anymore at this point, you can’t access its properties. At this stage, all the event listeners have been removed before the Vue instance is unmounted. So, this hook can be useful for tasks such as logging or communicating with other components.
In our current setup, it’s the ScoreComponent that handles the tasks of displaying and updating the score. We also have a parent component called App.vue with a button to manage the visibility of the ScoreComponent.
The destroyed lifecycle hook is called when the ScoreComponent is completely removed from the DOM. This includes the removal of its event listeners and the watchers, which happens after a user clicks the “Hide Score Component” button.
We can observe a log message in the destroyed hook confirming the destroyed component, indicating the end of that particular Vue instance lifecycle. We can also use this hook to clean non-Vue resources or to confirm that the component was removed to debug the app. The destroyed hook, along with the beforeDestroy hook, handles the teardown process for Vue components.
The parent component App.vue remains the same but the ScoreComponent.vue will look like the following:
<template> <div> <h1>Runs Scored</h1> <p id="score">Current Score: {{ runs }}</p> <button @click="singleRunTaken">Single</button> </div> </template> <script> export default { name: 'ScoreComponent', data() { return { runs: 0, }; }, methods: { singleRunTaken() { this.runs++; }, }, beforeDestroy() { console.log('Before Destroy Lifecycle Hook'); console.log('Final chance to access the DOM'); }, destroyed() { console.log('Destroyed Lifecycle Hook: Component has been destroyed'); } }; </script> |
6. Understanding Debugging Hooks
The hooks in this category allow the developers to identify and debug errors in real-time, both in the development and production environment.
6.1 onErrorCaptured Hook
The onErrorCaptured hook is called every time an error is detected in the child component. It logs and shows the error to the user as a part of the error-handling process. This lifecycle hook can catch errors related to the watchers, event handlers, life cycle hooks, and more from a Vue component in real time.
App.vue
<template> <div id="app"> <LifeCycleComponent /> </div> </template> <script> import { onErrorCaptured } from 'vue'; import LifeCycleComponent from './LifeCycleComponent.vue'; export default { name: 'App', components: { LifeCycleComponent }, setup() { onErrorCaptured((err) => { console.log('Error captured:', err.message); return false; }); } } </script> |
LifeCycleComponent.vue
<template> <div> <h1>Error Handling Component</h1> <button @click="throwError">Throw Error</button> </div> </template> <script> import { defineComponent } from 'vue'; export default defineComponent({ name: 'LifeCycleComponent', setup() { const throwError = () => { throw new Error('Something went wrong!'); }; return { throwError }; } }); </script> |
Registered in the App.vue setup function, we have the onErrorCaptured hook listening to its child components in case they emit any errors. When the throwError function throws an error in the LifeCycleComponent, the job of the onErrorCaptured hook is to capture the error and log an error message in the console.
To prevent an error from spreading across the component tree, it returns false. It provides a graceful method of handling errors, particularly those that are restricted to specific component hierarchies. This hook helps Vue developers in applying custom logic for error management and maintaining the stability of the application.
6.2 onRenderTracked Hook
When you set a render function to track or monitor a reactive component, the onRenderTracked hook is triggered. It starts running as soon as the reactive component is initialized. This debugging tool is called every time a reactive dependency is tracked.
6.3 onRenderTriggered Hook
Whenever a change is detected in the reactive component, the onRenderTriggered hook is called. It triggers a new render to update the display with the latest data. It is used during development as a debugging tool and is triggered when a reactive dependency causes the Vue component to rerender.
Here in Vue 3, we have two hooks called onRenderTracked and onRenderTriggered. These hooks are used to track reactive dependencies and component rendering events, respectively. In this component, there are two buttons that trigger changes, allowing the component to display and update the score and wickets.
The onRenderTracked hook captures reactive data during re-rendering. Whenever a tracked dependency is accessed, this hook logs the event details to the console.
On the other hand, the onRenderTriggered hook also captures the reactive data during re-rendering. If there are any changes to the values of score or wickets, it logs the event in the console along with the updated state of the component.
<template> <div id="app"> <p>Score: {{ score }}</p> <p>Wickets: {{ wickets }}</p> <button @click="singleRunTaken">Single Run</button> <button @click="wicketTaken">Wicket!</button> </div> </template> <script> import { ref, onRenderTracked, onRenderTriggered } from 'vue'; export default { name: 'RenderTracked', setup() { const score = ref(0); const wickets = ref(0); const singleRunTaken = () => { score.value++; }; const wicketTaken = () => { wickets.value++; }; onRenderTracked((event) => { console.log('event from render tracked', event); }); onRenderTriggered((event) => { console.log('event from render triggered', event); }); return { score, wickets, singleRunTaken, wicketTaken }; } }; </script> |
7. Conclusion
This article explored various hooks used at different stages of a Vue instance’s lifecycle. We examined how using these hooks can enhance the quality of the Vue apps by helping manage the behavior of Vue components effectively.
Vue developers can use these hooks to organize their code and perform specific actions at different stages of a component’s lifecycle. Knowing when and how to use Vue lifecycle hooks is essential for creating responsive and dynamic web apps.
FAQs
What parts of the Vue lifecycle hooks are executed during SSR?
Only the serverPrefetch lifecycle hook is implemented during SSR because it is used to fetch data from the server.
Is a lifecycle hook for both directive and component?
Yes, lifecycle hooks can be used for both directives and components. You can leverage its methods to tap into the key events of a directive or component to initiate change detection, create new instances, respond to updates, and perform cleanup after deleting instances.
Comments
Leave a message...