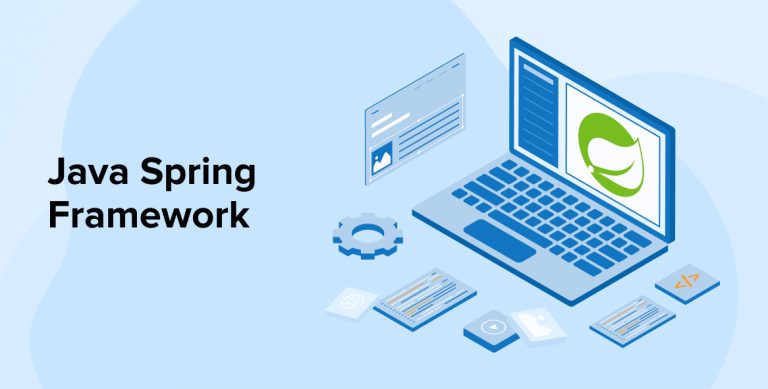
Java programming language is used to build a wide range of software solutions. It offers a myriad of tools and technologies to streamline the development process. One notable framework is Spring, which is popular for building web apps. Unlike Struts and other frameworks, it allows developers to create any layer of an application.
Leveraging this framework, a Java development company can easily deliver high-performance applications. Spring is quite popular in the Java community because it provides a large array of features. Let’s understand what this framework is and how to use it for development.
1. Overview of Java Spring
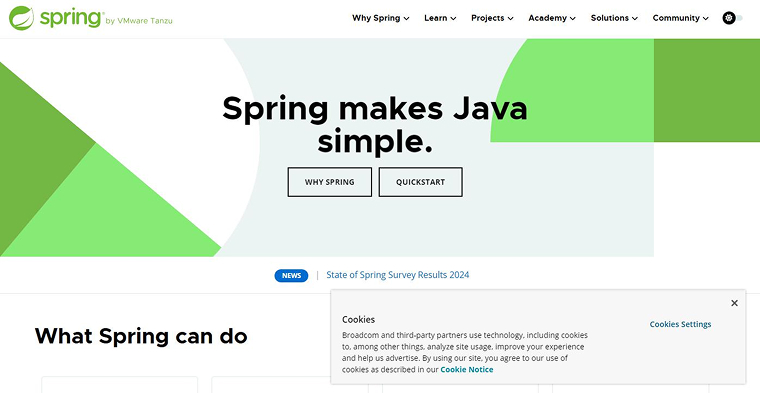
Spring is a robust and popular Java framework that simplifies the app development process. It offers a comprehensive infrastructure and Plain Old Java Objects (POJOs), enabling developers to build high-quality solutions ranging from web applications to enterprise-grade software. Using Spring, developers can significantly reduce the number of configuration tasks, which helps develop robust web apps.
It is a secure and flexible Java framework that enables developers to use resources efficiently. Spring also encourages you to write clean, secure, and more efficient code.
2. Advantages of Spring Framework
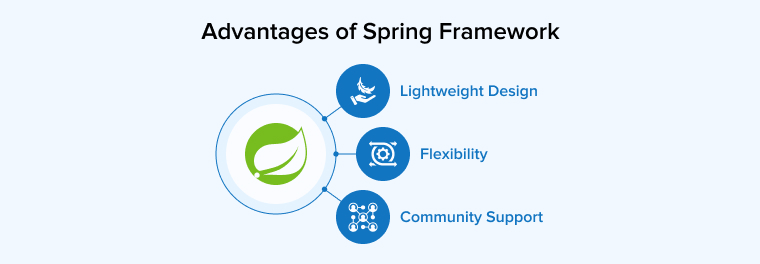
Opting for the Spring framework during Java development brings in a series of advantages such as:
2.1 Lightweight Design
Spring is often described as lightweight, but this doesn’t mean it has a smaller codebase, a small .jar file, etc. The term lightweight here refers to the fact that Spring has a minimal impact on your existing application. You don’t need to make significant changes or adjustments to your existing application to move it to Spring.
We don’t need any significant changes if you decide to use Spring for your Java application. The implementation of POJO also keeps the framework lightweight. Because with POJO on your side, you don’t need any additional interfaces.
2.2 Flexibility
The Spring framework consists of a modular architecture, allowing Java developers to pick and integrate only the components they require for the applications. Spring is an ideal option when working on projects with specific needs or requiring a custom setup. This Java framework is capable of handling everything from a simple web app to complex enterprise software.
2.3 Community Support
Spring has support from a large and active community. When developers are stuck with a problem, they can seek advice and necessary help to find solutions from the community on online platforms such as Stack Overflow.
A lot of tutorials and resources are available online to learn Java best practices and troubleshoot common challenges. Developers and businesses alike can attend events and meetups on the community page of its official website.
3. Spring Framework Architecture
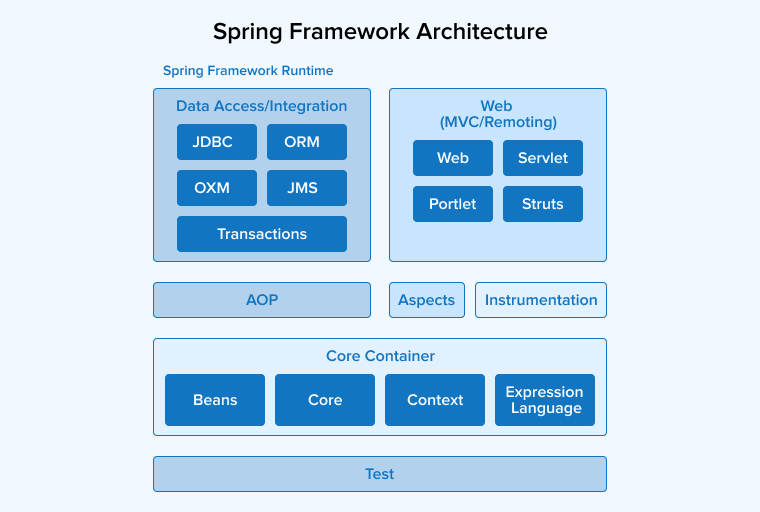
The Spring framework provides a range of modules to streamline Java app development. In this section, we will break down the architecture to understand every aspect and module of this framework.
3.1 Core Container
The Core Container offers the ApplicationContext, the Inversion of Control (IoC) container, and other basic functionalities of the Spring framework. It has various modules including:
- Spring Core: It offers the Spring framework’s fundamental functionalities such as Inversion of Control (IoC) and dependency injection. The IoC container helps create and handle instances of JavaBeans. It wires the beans together using dependency injection.
- Spring Beans: This module provides BeanWrapper, which allows developers to handle a bean’s life cycle. It also offers BeanFactory, which is the IoC container’s basic building block and a core interface to access IoC containers. This module offers methods and techniques to retrieve beans.
- Spring Context: You get an advanced version of the BeanFactory, i.e. the ApplicationContext from this module along with some additional features like the ability to consume and publish events, resource loading, and support internationalization.
- Spring Expression Language (SpEL): It’s a robust expression language that helps you query and manipulate objects during runtime. This module supports a rich set of features like type conversion, loops, conditionals, method invocation, and property access. It also allows you to access functions and variables defined in the application context and offers support for defining custom variables and functions.
3.2 Spring Framework Data Access
This component of the Spring framework is known as the Data Access/Integration container which includes the JMS, OXM, ORM JDBC, and the Transactions module.
- JDBC: With an abstract layer of JDBC, developers don’t have to register any monotonous code associated with the database manually.
- ORM: This module enables seamless integration with popular ORMs like JDO and Hibernate, which are implementations of JPA.
- OXM: Java developers can use this module to link the Object/XML – XMLBeans, JAXB, etc.
- JMS: It helps create send and receive messages.
- Transactions: All the transactions for classes implementing particular POJOs and other methods are handled by this module.
3.3 Spring Web
This layer consists of modules empowering the Spring framework’s web-based functions.
- Web-Portlet: Also known as the Spring-MVC-Portlet module, it can copy all the functionalities from the Web-Servlet module and provides Spring-based Portlets.
- The Web Module: Provides basic web-based application context, multipart file-upload functionality, and web integration features. It also offers servlet listeners to initialize IoC containers.
- AOP: It’s a language that programmers can use to add enterprise functionality to Java apps.
- Web-MVC Module: This web layer allows you to implement MVC and REST for Java-based web apps.
- Instrumentation: Offering loader implementations and class instrumentations, this module is utilized for specific app servers.
- Test: It supports testing tools like JUnit and TestNG to test Spring components. The module also allows you to load ApplicationContexts and cache them.
- The Web-Socket Module: Supports two-way communication between client and server in all Java applications.
4. Drawbacks of Spring Framework
There are indeed multiple benefits of using the Spring framework for app development. However, implementing Spring in Java development brings its fair share of challenges. Some of them are as mentioned below:
4.1 Parallel Mechanism
Although offering a wide range of options is considered an advantage, it can create confusion for developers about picking the right one. This may cause delays in projects and sometimes leads to incorrect decisions that negatively impact both the project and the business.
4.2 Configuration Complexity
Using Spring configurations can be a complex process, especially when it utilizes XML-based configurations. The bigger the size and complexity of a project, the more challenging it becomes to handle configurations. On the bright side, Spring does offer automated configuration options like Spring Boot and JavaConfig to remove some complexities from the configuration.
4.3 Fewer Guidelines
Spring provides proper documentation to guide developers on handling vulnerabilities like XSS and cross-site request forgery attacks. However, it still leaves room for many potential security issues. The lack of clear guidelines not only poses a challenge to the efficient use of the framework but also discourages developers from using it altogether.
5. Spring Framework Environment Setup
In the below section, we’ll discuss all the steps with proper commands to set up JDK, Tomcat, and Eclipse on your system.
Step 1 – Setup Java Development Kit (JDK)
Download the latest Software Development Kit (SDK) version from the official Oracle Java site. Go through the instructions in the downloaded files to install and configure the Java Development Kit (JDK). Set the environment variables, PATH, and JAVA_HOME to refer to the directory consisting of JAVA and JAVAC, usually java_install_dir/bin and java_install_dir, respectively.
Users operating the Windows OS will see the installed JDK in the C:\jdk1.6.0_15. Open the C:\autoexec.bat file and include the following lines:


If you’re using the Windows NT/2000/XP, right-click on My Computer, and select Properties → Advanced → Environment Variables. With this, you’ll be able to update the PATH value. Once, it gets updated, click on OK.
On Unix systems such as Solaris, and Linux you’ll find the SDK in /usr/local/jdk1.6.0_15. Open the C shell and insert the following command in the .cshrc file.


If you’re using Integrated Development Environments (IDEs) such as IntelliJ IDEA, Eclipse, Sun ONE Studio, or Borland JBuilder, start by writing a basic Java program. Then, compile and run it. This process will help your IDE recognize the location of the installed Java file. If needed, refer to the downloaded documentation to implement the setup properly.
Step 2 – Install Apache Common Logging API
Download the latest version of Apache Commons Logging API from https://commons.apache.org/logging/. After successful download, unpack the binary distribution at your desired location. For example, in Windows, it’s C:\commons-logging-1.3.4, while on Linux/Unix, you can use/usr/local/commons-logging-1.3.4. The below image depicts the jar files and other supporting documents in this directory.
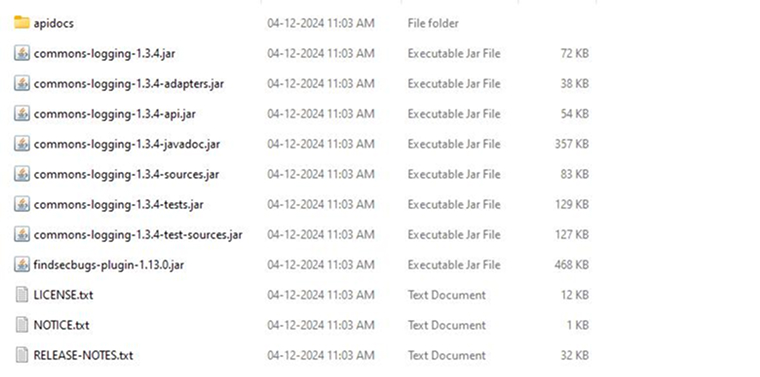
Check that the CLASSPATH variable is set properly on this directly otherwise, there will be issues while running the application.
Step 3 – Setup Eclipse IDE
We recommend using the latest version of the Eclipse IDE to do the coding in the Spring framework. Download the latest version from https://www.eclipse.org/downloads/. After downloading, extract the binary distribution at your preferred location, such as in C:\eclipse on Windows or /usr/local/eclipse on Linux/Unix. Finally, set the PATH variable. In Windows, double-click on the eclipse.exe or execute the below commands to start Eclipse.

For Unix OS like Linux and Solaris, start the IDE using the following

You will see the below Eclipse Home page on your screen on the successful start of the IDE.
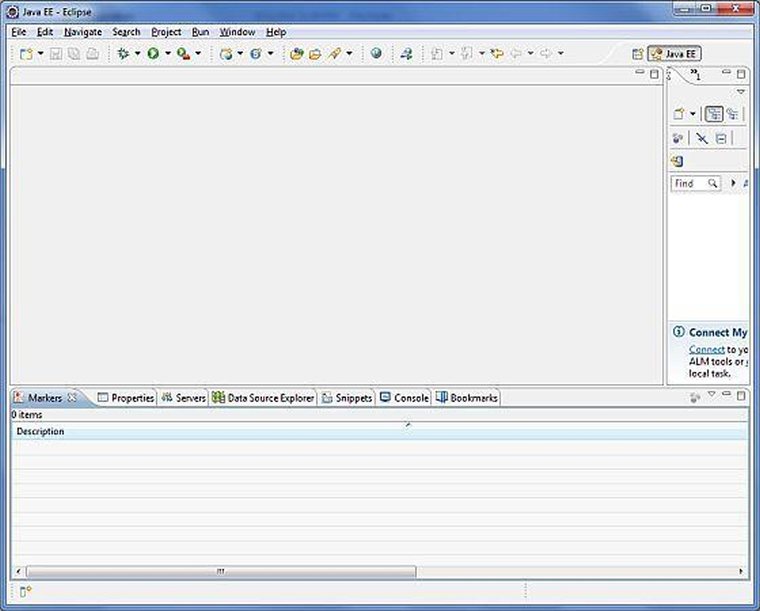
Step 4 – Setup Spring Framework Libraries
We’ll move towards the Spring framework setup if the above steps are successfully executed on your system.
To install the Spring framework, follow the below steps sequentially.
- Download the .zip file if you’re a Windows user or .tz file using a Unix-type OS.
- Download the latest version of the Java Spring framework from https://repo.spring.io/release/org/springframework/spring.
- Extract the files from the zip archive.
The E:\spring\libs directory consists of all the Spring libraries. Therefore, ensure that you set the CLASSPATH variable properly, otherwise, to avoid issues when running the app, make sure to set the CLASSPATH, as it is configured by default.
Make sure that all the steps are executed as directed in this installation guide.
6. How Does Spring Framework Work?
The Spring Framework primarily functions based on two fundamental principles: Dependency Injection (DI) and Inversion of Control (IoC).
6.1 Inversion of Control (IoC)
IoC is the reversal of the program’s control flow. It is a design principle where the control of objects or portions of a program is transferred to a container or framework, rather than being controlled by the program itself.
The IoC Container’s Role in Spring:
The IoC container in Spring is responsible for creating, managing, and injecting the dependencies of beans. It serves as the core of the Spring Framework and facilitates the wiring of the application’s components together.
Types of IoC Container:
1. BeanFactory:
Beanfactory delays the creation of beans (lazily initializes them) until they’re needed and generally used for lightweight applications with minimal requirements.
import org.springframework.beans.factory.BeanFactory; import org.springframework.beans.factory.xml.XmlBeanFactory; import org.springframework.core.io.ClassPathResource; import org.springframework.core.io.Resource; public class BeanFactoryExample { public static void main(String[] args) { Resource resource = new ClassPathResource("beans.xml"); // Load bean definitions from XML BeanFactory factory = new XmlBeanFactory(resource); // Create BeanFactory // Get a bean from the container MyService myService = (MyService) factory.getBean("myService"); myService.doSomething(); } } |
In the above example, BeanFactory is used to load bean definitions from an XML file (beans.xml). It lazily loads the beans when they are requested using getBean().
2. ApplicationContext:
The ApplicationContext eagerly loads beans during startup and provides additional features like event handling, internationalization support, and more.
import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class ApplicationContextExample { public static void main(String[] args) { // Load the context and beans from the XML file ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml"); // Get the bean from the container MyService myService = (MyService) context.getBean("myService"); myService.doSomething(); } } |
In the above example, ApplicationContext is used, which eagerly initializes the beans during startup and provides more features compared to BeanFactory.
6.2 Dependency Injection(DI)
Dependency Injection (DI) is a design pattern that helps promote loosely coupled code by injecting dependencies from an external source rather than creating them within the class.
Importance of Dependency Injection(DI):
Loose Coupling: DI promotes loose coupling between objects. Instead of classes being responsible for creating their dependencies, these dependencies are injected into the classes. This approach makes it easier to change or replace components without affecting others.
Testability: Dependency Injection makes testing easier, as you can inject mock dependencies into classes for unit testing.
Maintainability: By decoupling components, DI makes the system more flexible and maintainable.
Types of Injection:
There are three methods to inject dependencies in the Spring framework:
1. Constructor Injection:
@Component public class Car { private Engine engine; // Constructor Injection public Car(Engine engine) { this.engine = engine; } } |
2. Setter Injection:
Dependencies are provided via setter methods after the object is constructed.
@Component public class Car { private Engine engine; // Setter Injection public void setEngine(Engine engine) { this.engine = engine; } } |
3. Field Injection:
Dependencies are injected directly into fields (also called property injection).
@Component public class Car { @Autowired private Engine engine; // Field Injection } |
7. Conclusion
This article sheds some light on one of the most popular, versatile, and robust Java development frameworks called Spring. It highlights the framework’s benefits and limitations, offering a comprehensive understanding of its underlying components.
This guides you through the process of downloading, installing, and setting up the Java Spring framework for development purposes. No matter if you are just starting or want to take your existing project to another level, this framework can certainly be useful to you.
Comments
Leave a message...