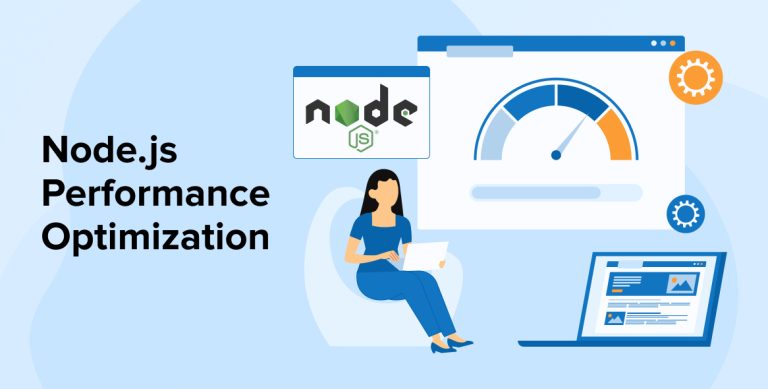
When creating web applications, performance should be a top priority. Among the many popular and flexible backend technologies, Node.js has long been a go-to for programmers looking to build scalable, high-performance programs. But, like with any other technology, there are obstacles to overcome while working with Node.js.
To help you grow your Node.js applications, this post will go over several development tips to achieve the best Node.js performance. Then, even very heavy workloads won’t be a problem for your application.
You can give your software product the performance boost it requires to be successful in the market by hiring the best NodeJS development company. They’ll use all tried-and-tested techniques to enhance the performance metrics provided in this post.
1. Top Tips to Consider For Node Js Performance
Thanks to its rapidity, scalability, and seamless user experience, Node.js has become a popular runtime environment for the globally used programming language Javascript. Although, the speed of Node.js programs can grow problematic as they expand in size and complexity.
Some tried-and-true methods for improving performance and making your Node.js application faster and more scalable are as follows:
1.1 Reduce Latency Through Caching
Server-side caching is a common tactic to improve a web app’s speed. Its primary objective is to speed up data retrieval by reducing the time spent calculating data or performing I/O operations, including getting data from a database or via a network.
For frequently accessed data, there is a high-speed storage layer called a cache that may temporarily store it. Only sometimes you need data, you have to go to the main source, which is often slower.
When dealing with data that doesn’t undergo frequent changes, caching is the way to go. Caching data will likely improve your web application performance if it gets several requests for the same unmodified data.
Caching the results of computationally challenging tasks is also an option, provided that the cache can be utilized for subsequent requests. This keeps the server resources from being overloaded with unnecessary computations of this kind.
Caching is also commonly used for API requests to other systems. Take it for granted that the answers are safe to use again for similar queries. It is advisable to keep API calls in the cache layer to avoid extra network queries and other expenses related to the API at hand.
One easy way to set up caching in a Node.js application is via an in-process caching solution, like node-cache. It entails making data that is utilized often more quickly retrievable by keeping it in memory.
The main issue with an in-process cache is that it can only be used by one application process at a time. This makes it ill-suited for distributed workflows, particularly when storing items that can be changed.
Redis and Memcached are two examples of distributed caching solutions that might be useful in such cases. When a program is scaled across many servers, it runs by itself and becomes more effective.
1.2 Optimizing Data Handling Methods
Performance relies on optimization, which streamlines system operations and increases the app’s overall efficiency generally. Is there anything specific about a Node.js application that could benefit from optimization? Begin by examining the current state of data handling. A CPU/IO-bound operation, such as a database query or API request, might cause Node.js apps to run slowly.
The majority of Node.js apps get their data by sending an API call and receiving a response. Is there a way to make it optimum? Pagination is a typical technique; it involves dividing replies into batches of material and making those batches accessible through selected response requests. Using pagination, you may optimize the response while still passing more data to the user’s client.
The ability to filter results according to the requester’s criteria is another successful method. This not only allows customers to fine-tune the provision of resources according to their needs, but it also decreases the total number of calls and the results given. In the architecture of REST APIs, these two ideas are prevalent.
The process of fetching data is related to underfetching and overfetching. The first one gives the client more information than they need, whereas the second one doesn’t provide them enough information and frequently needs a callback function to another endpoint to finish collecting data. Inadequate app scalability can lead to these two issues, which can arise on the client side. By using GraphQL, the server is spared the guesswork and delivers the exact data that the client requested. This makes it ideal for problems like these.
1.3 Load Balancing
One typical difficulty is developing fast apps that can manage a high volume of incoming connections. Distributing the traffic to equalize the connections is a common approach. This method is known as load balancing. You can manage additional connections with ease using Node.js’s ability to replicate application instances. Either one or more multicore servers can handle this.
Using the newly introduced cluster module, you can expand your Node.js app on a multicore server by creating several worker processes one for each CPU usage core that link to one master task and share the server port. That is how it operates, much like a large Node.js server with many threads. The cluster module can be used to enable load balancing and allocate receiving connections with a round-robin approach between all the employees using numerous CPU cores in an environment.
const cluster = require('cluster'); const http = require('http'); const numCPUs = require('os').cpus().length; if (cluster.isMaster) { for (let i = 0; i < numCPUs; i++) { cluster.fork(); } } else { http.createServer((req, res) => { res.writeHead(200); res.end('Hello World\n'); }).listen(8000); } |
Code Explanation: In this example, we handle incoming requests by forking multiple worker processes using the built-in cluster module. NodeJS can make the most out of multicore systems because every worker here processes the requests.
The PM2 process manager is another option for permanently preserving applications. In the event of a code update or problem, this helps to keep the program up and running by reloading it. It is not necessary to modify your code to use the native cluster module because PM2’s cluster functionality allows you to run several processes over all cores.
There are problems with the current single-cluster arrangement, thus we should be ready to move to a multi-server design that uses reverse proxying for load balancing. NGINX is compatible with several load-balancing techniques and numerous Node.js servers, including:
- Round robin – It means that each server in a list gets visited once fresh requests are made.
- Least connections – The server with the fewest active connections is the one that receives new requests.
- IP hash – The IP address is hashed so that a fresh request can be sent to the server associated with it.
With the reverse proxy capability, you can run several web application servers with ease and insulate the Node.js server from direct involvement with web traffic.
1.4 Use the Cluster Module to Scale the Server
Asynchronous code execution in the background using multiple threads is made possible in Node.js, a single-threaded language. Programs written in Node.js are unable to make full use of a multicore processor.
In rare cases, recessions, delays, or outages may occur because a surge in incoming traffic only loads one core. Clustering is a great tool for scaling Node.js performance in this situation.
With Node.js clustering, several child processes can use a single server port and execute in parallel, each with an independent event loop. In this approach, a single machine can handle horizontal scaling of the Node.js server.
Clustering makes server scalability straightforward, so you can increase Node.js application performance anytime there’s a lot of traffic. In this approach, cluster modules in Node.js apps may guarantee ROI while keeping businesses running smoothly.
1.5 SSL/TLS and HTTP/2
Using HTTP/2 while developing Node.js web applications can reduce bandwidth use and significantly improve online surfing speed and ease. The main goals of HTTP/2 are to fix problems with HTTP/1.x and to increase performance. Examples of setting up an HTTP/2 server.
const http2 = require('http2'); const fs = require('fs'); const server = http2.createSecureServer({ key: fs.readFileSync('server-key.pem'), cert: fs.readFileSync('server-cert.pem') }); server.on('stream', (stream, headers) => { stream.respond({ ':status': 200, 'content-type': 'text/plain' }); stream.end('Hello HTTP/2!\n'); }); server.listen(3000); |
Code Explanation: The above code snippet shows how you can use Node.js to create a secure HTTP/2 server. It provides features like multiplexing and header compression that improve NodeJS performance.
Included in HTTP/2’s features are:
- Header compression – By default, all HTTP headers are compressed before being sent, and this feature eliminates any extraneous ones.
- Multiplexing – It refers to the practice of allowing several requests to share a single TCP connection and its associated resources and response messages.
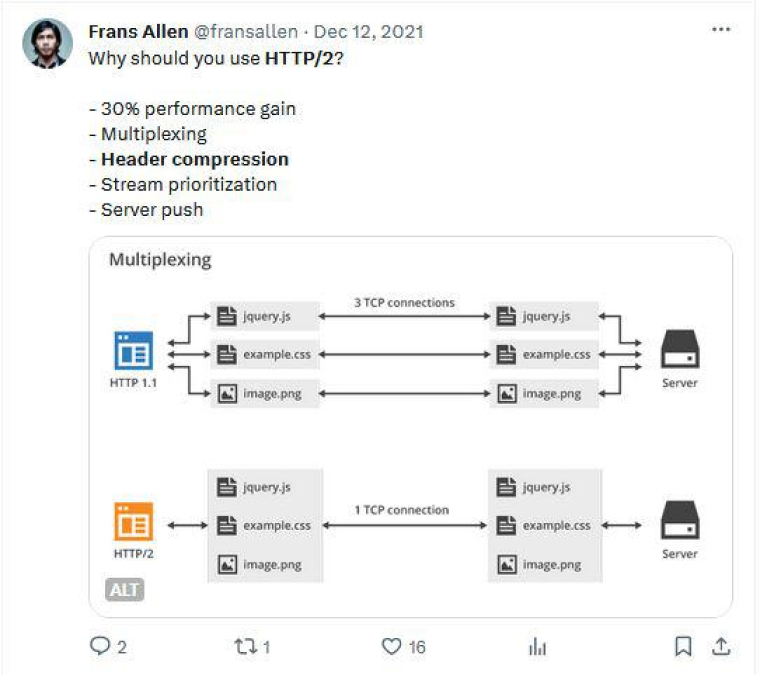
Reducing the amount of server requests is the main objective of multiplexing. When sending data over HTTP, the time spent establishing the connection might easily outweigh the actual data transmission time. Secure Sockets Layer (SSL) and Transport Layer Security (TLS) must be implemented to use HTTP/2. An HTTP/2 server can be quickly and easily set up using this version of Node.js.
1.6 Using WebSockets For Effective Server Communication
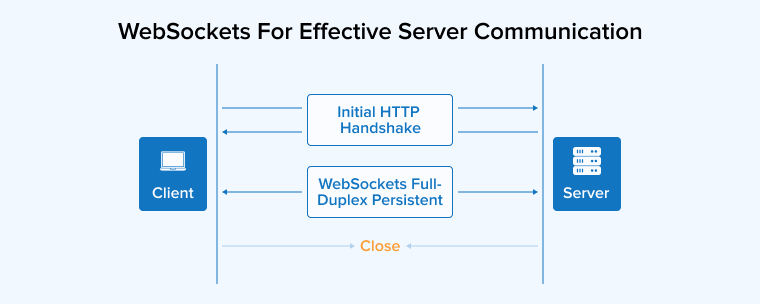
The Hypertext Transfer Protocol (HTTP) has been the foundation of the Internet since its inception. WebSockets allow web apps to communicate without using HTTP. They enable the client and server to communicate in both directions for an extended period. The channel remains open once created, providing persistent and fast communication between the client and the server. Low latency and overhead allow both parties to begin sending data whenever they choose.
For infrequent data exchange and client-driven communication including user engagement, HTTP is useful. By using WebSockets, both the server and the client may communicate in real-time without the need for the client to formally request it. Both short-term and long-term conversations benefit greatly from this.
To build a WebSockets server, many Node.js developers turn to the widely-used WebSockets library. To listen for events on a server that supports WebSockets, the front end uses JavaScript to connect to the server. With WebSockets, you can achieve a high-competition architecture without sacrificing speed, which is essential for concurrently holding multiple requests.
Setting up a WebSocket server using the ws library. const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 8080 }); server.on('connection', (socket) => { socket.on('message', (message) => { console.log(`Received: ${message}`); socket.send(`Hello, you sent -> ${message}`); }); }); |
Code Explanation: Using the above code, we can create a WebSocket that can listen to incoming connections. It helps both client and server to communicate in real time as they no longer require a request-response cycle to send messages.
1.7 Memory Management
If you want to avoid excessive usage of memory or memory leaks in your NodeJS app then you have to make sure that efficient memory management is in place. It reduces the time spent after memory allocation and garbage collection which helps improve the response time and performance of your app.
Effective memory management also enables you to scale your NodeJS app and manage more concurrent users. It also helps minimize your application’s overall memory footprint.
1.8 Employ Scaling Strategies
Calibrating the capacity of your web app to meet the changing or increasing load and performance requirements is called the scaling process. You can accomplish scaling in the NodeJS app using the following strategies:
- Horizontal Scaling: When you reduce the load on a single server by adding more machines or instances, it is called horizontal scaling. Here, every server would be managing a small part of the total workload. This helps improve the performance and increase the capabilities of your application.
- Vertical Scaling: When you upgrade resources like memory and CPU to increase their capacities, it is known as vertical scaling. This helps the server manage more load and offer enhanced performance.
1.9 Utilize a Content Delivery Network (CDN)
Effective implementation of a Content Delivery Network can significantly improve your app’s performance. CDN is a global network of servers that is capable of caching and delivering static content. This helps enhance the loading speed as well as the user experience. Here is a step-by-step process for the efficient use of a CDN.
- Choose a CDN provider: Select a suitable CDN provider such as Amazon CloudFront or Cloudflare.
- Integrate your app with CDN: You have to modify your DNS setting and configure certain cache rules to integrate the CDN with your NodeJS application.
- Serve static content from the CDN: The loading speed will increase as you reduce the load on the server by serving JavaScript files, stylesheets, images, and other static content from the CDN.
- Monitor CDN performance: To ensure the safe and efficient delivery of your content, you have to keep an eye on your CDN. It also helps find performance issues at early stages.
2. Conclusion
This tutorial went over how Node.js speed is improved by HTTP/2, various caching techniques, and data handling approaches while aiming for a successful web application development. Afterward, we went over the steps to load balancing in a Node.js app, how to handle additional connections, the impact of stateful and stateless client-side security on scalability, and lastly, how to establish a solid connection between the server and client using WebSockets. You are now fully equipped to make use of Node.js performance optimization tactics and create user-friendly, efficient apps.
FAQ
How to increase NodeJS performance?
Follow these steps to increase NodeJS performance:
- Monitor & Measure Your Application Performance
- Reduce Latency Through Caching
- Optimizing data handling methods
- Load balancing
- Use the cluster module to scale the server
- SSL/TLS and HTTP/2
- Secure Client-side Authentication
- Using WebSockets for effective server communication
How efficient is NodeJS?
Node. js’s compact, successful, event-driven, and non-blocking I/O style allows for a fast execution pace. It depends on three coding traits that are—Async, Concurrency, and Parallelism—to ensure performance.
Is NodeJS high-performance?
Chrome’s V8 JavaScript engine, upon which NodeJS is based, converts scripts written in JavaScript into machine code. Yes, this is how Node.js can process a flood of http requests without slowing down. A few reasons why Node.js is great for creating fast web apps are these:
- Asynchronous I/O Model
- Event-Driven Design
- JavaScript’s execution rate
- Cache Functionality
- Streaming Data Accessibility
Comments
Leave a message...