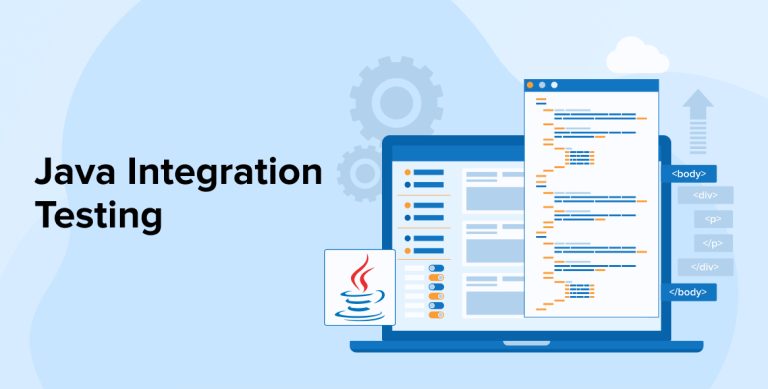
Java applications grow large and complex over time. The relationship and communication between components and services become more intricate. In such times, Java integration testing becomes essential.
Businesses must collaborate with Java development companies to ensure that all their systems operate up to expectations. These companies can help validate that all software modules and components are properly integrated before releasing the applications.
Integration testing is a new necessity in the era of continuous development and automated deployment. It helps maintain quality and minimize future technical debt. This article explores tools and best practices for effective Java integration testing.
1. What is Java Integration Testing?
Java integration testing is about checking whether different components of your Java code can work together as a unified application without any difficulty. The purpose here is to identify potential integration issues that may hinder the functionality of your app. Therefore, developers leverage software testing tools to create various real-life scenarios under which the program is tested thoroughly, ensuring the delivery of reliable and resilient Java software.
2. Java Integration Testing Best Practices
When you think about implementing integration testing, keep the following best practices in mind:
2.1 Before Unit Testing, Run the Integration Test
The larger the delay in discovering bugs, the more costly they become to fix. So, to ensure a seamless development cycle, it’s essential to catch the errors before perfecting crucial components of your system. And that means conducting integration testing before unit testing.
Discovering that your units are not compatible with the services after you have perfected their code would be disappointing. Fixing these issues will be both overwhelming and time-consuming. So, it’s smart to conduct an integration test before running a unit test.
2.2 Know the Difference between Integration Testing and Unit Testing
It is important to know that both a unit test and an integration test serve different purposes and fail for different causes. Unit tests are isolated and don’t need any external resources. Meanwhile, an integration test requires additional components, like a network or database, to verify how different components can work cooperatively.
Unit test is to test the performance and functionality of a single unit, whereas the integration test is to check the compatibility of collaboration between two different units. Unit tests are small and simple, integration tests are more complex as they require different tools and infrastructure.
A failed unit test indicates the presence of a bug in the business logic. However, when the integration test fails, it indicates an issue with the environment that needs to be addressed.
2.3 Never Conduct an Integration Test and Unit Tests Together
You can’t check for integration when conducting unit tests, as both tests have different natures. Unit tests measure the internal quality, whereas integration tests check external connections. Mixing them would only increase confusion and become a hindrance to the progress of your project. Therefore, it is essential to conduct both tests separately.
2.4 Use Detailed Logging
Keep detailed logs while running an integration test. It is very easy to understand the reason for failure in unit tests because their scope is narrow. The same can not be said when the integration test fails.
As integration testing consists of multiple components, identifying the cause of the failure can be challenging. However, keeping a detailed log of the tests can help you solve this problem. Examining these logs can help you understand whether the problem lies in the code itself or external resources.
3. Top Java Integration Testing Frameworks
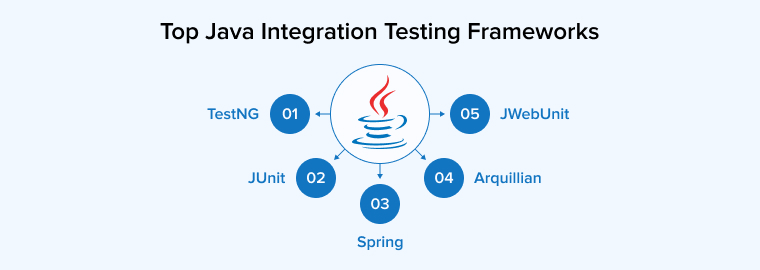
After discussing the best practices for integration testing, let’s have a look at the frameworks along with their pros and cons to help you determine which one is the suitable option for your project.
3.1 TestNG
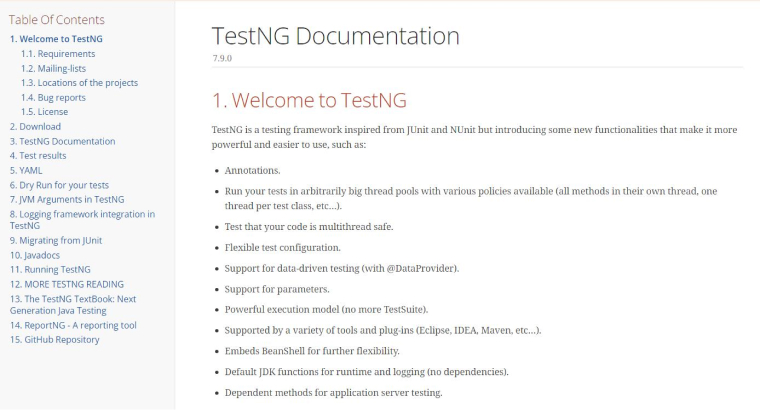
Created by Cedric Beust, TestNG is a test automation framework. Its design is influenced by JUnit and NUnit. The reason behind its popularity as one of the best Java testing frameworks is because of its advanced capabilities like parameterizing, grouping, and sequencing.
The QA team can leverage its superior functionalities and annotations to enhance the software testing process. With its advanced features, TestNG can easily overcome the limitations of earlier testing frameworks and adapt to new test cases.
Pros:
- Provides a group testing facility.
- Allows you to set a priority for test cases.
- Offers flexible runtime API plugins and runtime configurations.
Cons:
- It takes time to set up and install TestNG.
- It’s not very useful if you don’t have priorities for test cases.
- Requires certain experience and expertise which is hard to find.
3.2 JUnit
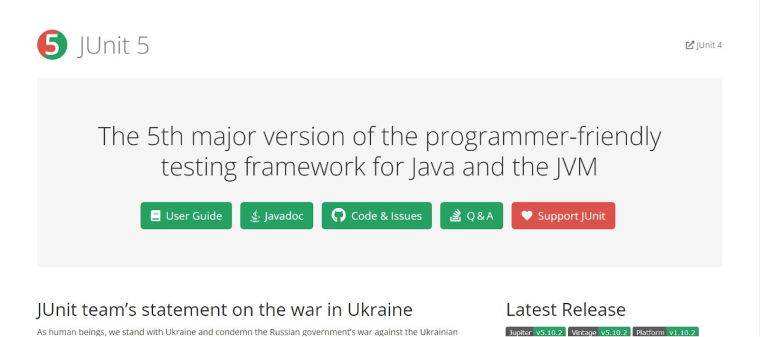
While JUnit is largely used for unit testing, it can also be used for integration testing. Let’s understand how. JUnit adopts a test-driven software development methodology. It follows a “test a little, code a little” technique.
When using this tool, each section of code is tested first to check whether it is written correctly. Only after its validation, a developer can move on to write another piece of code. Such a technique of writing code helps you ensure that it delivers the expected results.
It is used to test the code for all kinds of scenarios, enabling you to test the app or its components for integrations or compatibility with other components and services.
Pros:
- Increases the efficiency of the tests.
- Annotations help you save time.
- Enables you to retest all possible scenarios.
- Quick reporting
Cons:
- Doesn’t support group testing.
- Doesn’t support GUI testing.
- It does not offer any functionality for creating HTML reports after test completion.
3.3 Spring
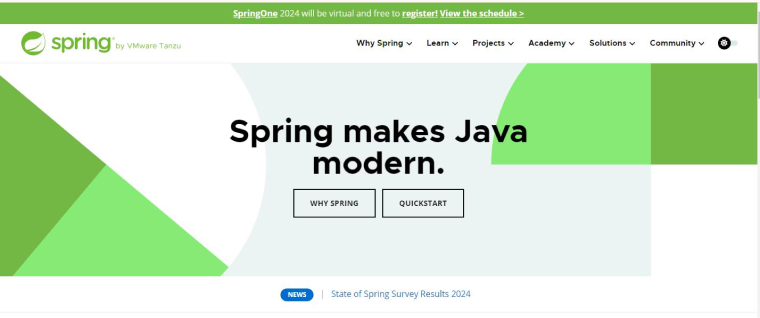
The Spring Test framework is a powerful yet lightweight Java integration testing framework. It allows developers to automate writing tests for spring applications, supporting both unit tests and integration tests.
Spring Test DbUnit is used to integrate the Spring Test framework with the DbUnit. Moreover, a Spring Test MVC HTMLUnit is used to integrate the Spring Test MVC framework with HTMLUnit. These tools not only simplify the software testing process but also increase its efficiency by automating it. This framework is largely used to automate unit testing and integration tests for Java applications.
Pros:
- Does not require a web server to start the app.
- It can test the app for backward compatibility.
- Supports XML configurations.
- Minimizes the errors when testing.
Cons:
- Steeper learning curve due to difficult programming methods.
3.4 Arquillian
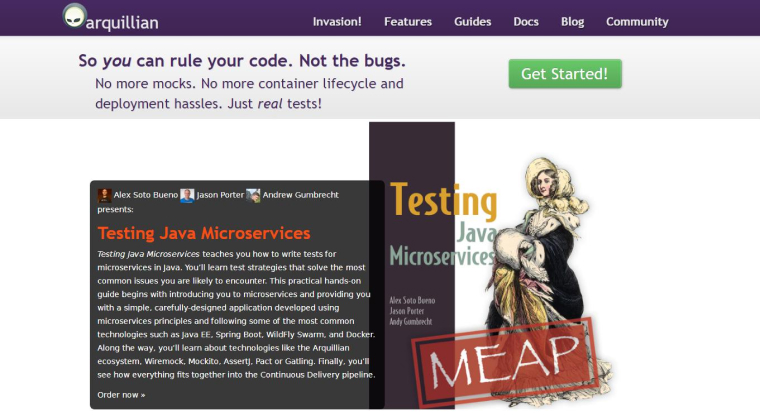
Arquillian a project from JBoss, effortlessly manages all the complexities of starting and stopping the containers. This allows developers to carry out Java integration tests without any hassle. Arquillian also supports app servers to enhance the developer experience by enabling them to manage the container lifecycle. This testing framework is specific to Java EE and needs very little time and resources to write integration tests for Java enterprise applications.
Pros:
- Provides seamless integration with TestNG and JUnit.
- Allows you to control the size of the package for testing deployment.
- Supports container management.
- Wraps everything for the test in the archive.
Cons:
- Creating test packages is time-consuming.
- Every test execution is deployed separately.
3.5 JWebUnit
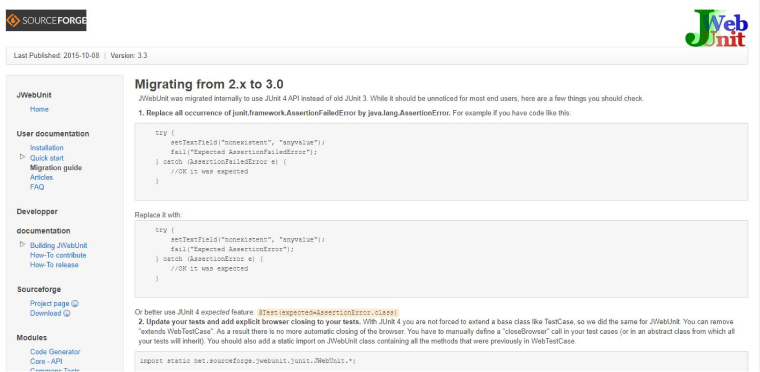
An extension of JUnit, JWebUnit is preferrable for functional testing, regression testing, and integration testing. This Java-based testing framework works with Selenium and HTMLunit, allowing you to test the accuracy of your application with a simple and easy-to-adopt test interface.
Pros:
- Ready-to-use assertions for rapid testing.
- Customized to test web applications.
- Tests the authenticity of the application.
Cons:
- The JS it uses for testing is complex.
- Can’t run on a headless server.
- Expensive as it needs experts for effective use.
4. Java Integration Testing Process
Here, we provide a step-by-step process for executing integration tests on Java applications.
- Define the Scope of the Test: First of all, know which components and modules need to work together in your Java app. This includes understanding the dependencies between different parts of the application.
- Prepare the Test Environment: Now, set up an environment where you can run integration tests without any interruption. Your testing environments must replicate the production environments as accurately as possible. This will be able to get accurate results.
- Develop Test Cases and Test Data: Create test cases that cover all the paths that data might take through the integrated components. If you have any prior test data that can help simulate the real-world usage of the application, then use them in the integration test.
- Use Integration Testing Tools: Employ tools and frameworks that support integration testing in Java. Tools like JUnit (for unit testing that can extend to integration testing), Mockito (for mocking external dependencies), and Arquillian or Spring Test (for more comprehensive integration testing) can be very helpful.
- Execute Tests: Next, run the tests as per your test cases. You can do it either manually or automate the tests, depending on the tools and techniques you use.
- Analyze Test Results: Examine the results of the tests to identify errors or issues with the integration of components. Look for failures, exceptions, and unexpected behavior that may have occurred.
- Fix Issues: Address any problems found during testing. This may involve correcting code in individual units or resolving issues with how components interact.
- Regression Testing: After fixing issues, perform regression testing to ensure that changes have not adversely affected other parts of the application.
- Repeat as Necessary: Integration testing is not a one-time process. With every addition of a new feature or an update, repeat the same testing process again and again. This will help maintain the compatibility and stability of your Java application.
- Documentation: Do not forget to document your entire integration testing process, including test cases, results, issues, and solutions. This documentation will be helpful in maintenance and future testing cycles.
5. Conclusion
Integration testing is a critical aspect of the software development lifecycle. It helps verify the interactions and collaboration between different systems, modules, and components. Using Java integration testing, you can find and fix issues arising during the integration process to ensure seamless performance.
The behavior, data flow, and interfaces of the integrated components are validated through integration testing. It helps discover faults and inconsistencies in different parts of the system during interactions or collaboration. Integration testing not only ensures the seamless function of an individual component, but also helps meet the reliability, security, and performance requirements of the entire system.
Additionally, there are many benefits of running integration tests like enhanced overall software quality, reduced integration risks, increased confidence in system behavior, improved system stability, early detection of integration errors, and more. Integration tests help find errors early in the development cycle, reducing their impact on the finished final product.
However, it is important to follow best practices and use the right tools for Java integration testing. These approaches can help overcome challenges along the way and simplify the process.
Comments
Leave a message...